I recently attempted to include a "Take Picture" functionality in my native mobile app, with the intention of storing the image as a base 64 encoded string. However, I encountered a challenge when I discovered that the existing "Take Picture" activity in Mendix only accepted the image as an object. To resolve this issue, I decided to customize the existing JavaScript action to incorporate the necessary code.
You can download the Module from the Mendix Market Place here,
https://marketplace.mendix.com/link/component/205786Introduction:
As I have explored various online forums, I have come across several discussions on the issue of synchronizing multiple image objects in native applications. In this scenario, a user visits a site and captures multiple images using the "TakePictureActivity." However, the synchronization process can be time-consuming as it takes massive amounts of time to store the images in the database.
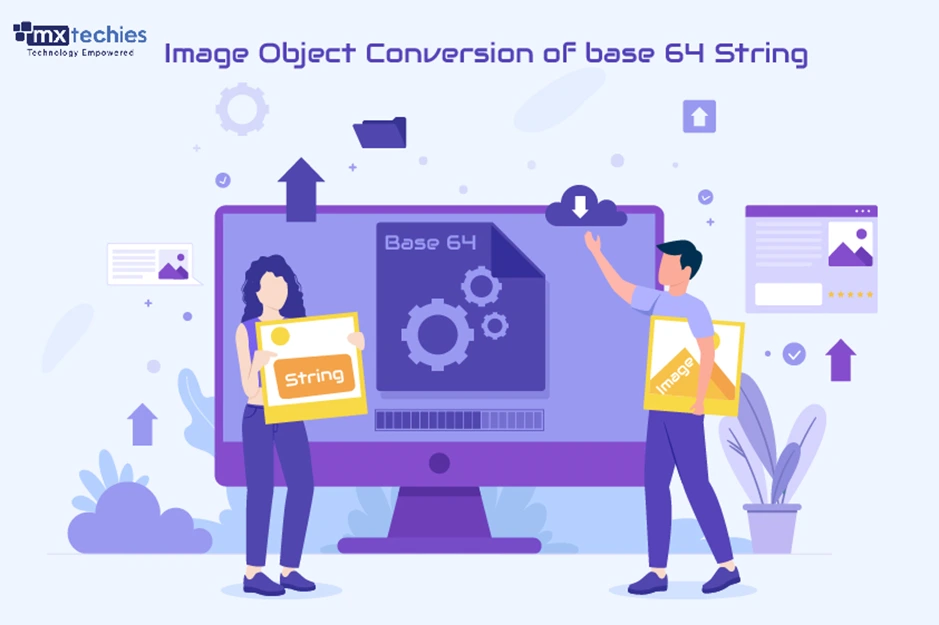
To overcome this challenge, we have developed an innovative solution to synchronize images as base64. By converting the images into base64 objects before committing them to the database, we can significantly reduce the synchronization timing and make it more efficient, even in low bandwidth networks.
Prerequisites:
How:
For each site entity, multiple images can be captured and stored in the "siteImages" entity. During the image capture process, the "TakePictureActivity" will be executed, and the images will be converted into base64 using the following code:
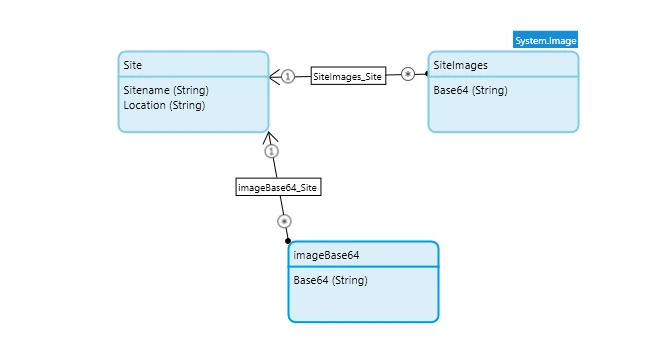
Code explanation:
Created an entity SiteImages and stored the base64 value in the Base64 attribute.
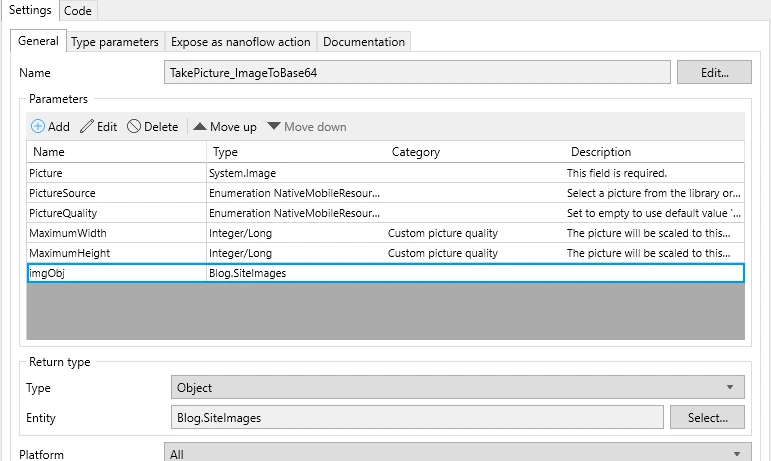
Created a new JavaScript action to handle the base64 content once a picture is captured.
- Added a new parameter
- Name: imgObj
- Type: object
Using react-native-image-picker library
- Creating variable imagebase64 and returning the stored file
- And added an imgObj on the export function
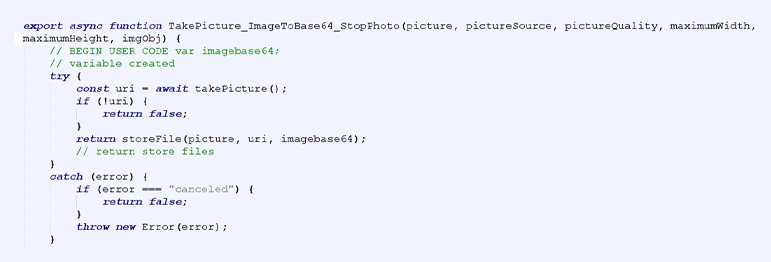
- Print the base64 working on the console log and the response on the console and store it in the created variable imagebase64 and check the response when a picture is not taken.
- Instead of returning an undefined variable replaced with a response
- For error handling.
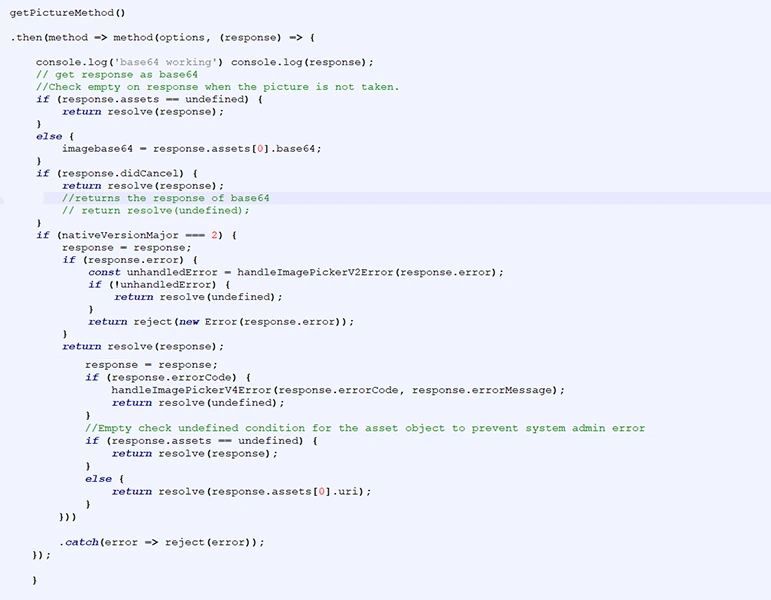
- Set the exact attribute name used on the siteImages entity
Eg:Base64
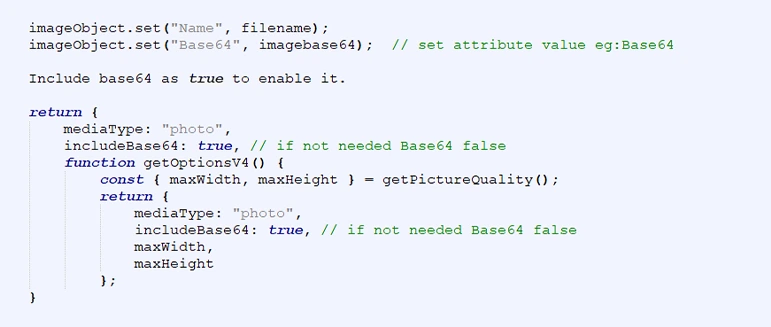
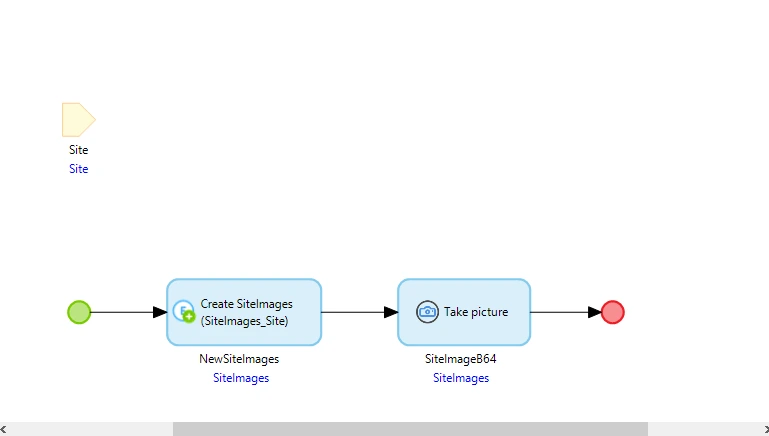
The siteImages entity now holds both images and their respective base64 values, however, due to the complexity of synchronizing both types of data, we have decided to only synchronize the base64 values. To achieve this, we have created a separate entity called imageBase64 to store the list of base64 values.
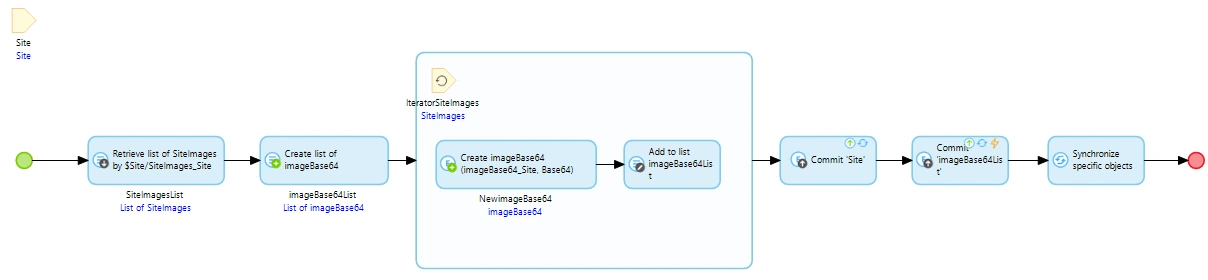
The siteImages entity is used to create a list of imageBase64 values, which is the only data that is synchronized. When it comes to committing the imageBase64 entity to the server, we have added a crucial step to the process. Before committing, an event handler is triggered to convert the imageBase64 values back into images, which are then committed to the siteImages entity and linked to the corresponding sites.
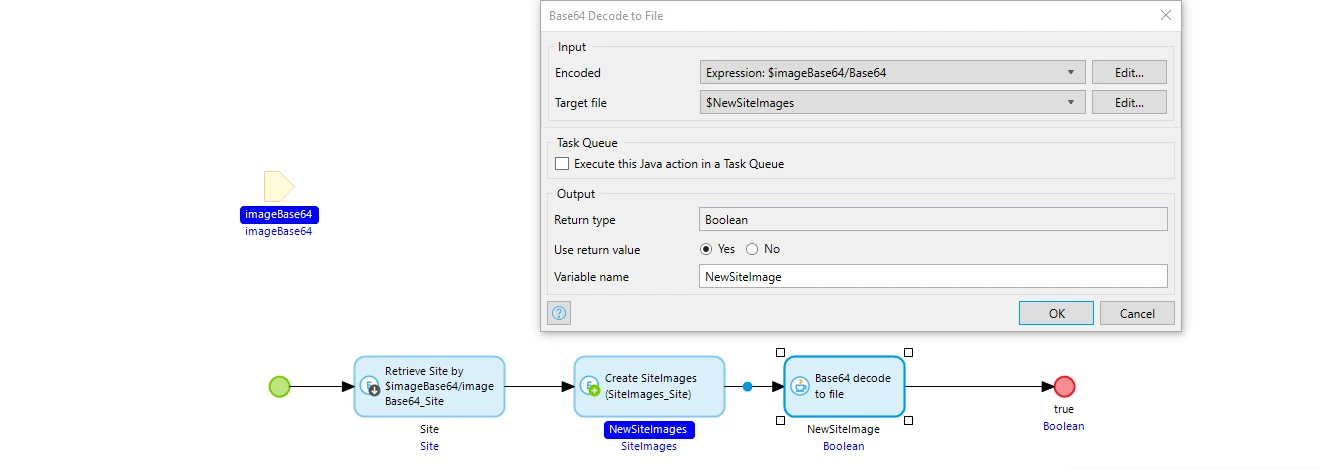
Finally, The above-mentioned methodology will optimize the synchronization process of large image files, ensuring that they are transferred as efficiently as possible even in low network conditions (2G or 3G). This will significantly reduce the time it takes to synchronize these large files.
Reference
https://docs.mendix.com/howto/extensibility/write-javascript-actions/
https://docs.mendix.com/howto/extensibility/write-javascript-github/
https://docs.mendix.com/howto/extensibility/best-practices-javascript-actions/
https://www.npmjs.com/package/react-native-image-picker
https://github.com/react-native-image-picker/react-native-image-picker